Introduction
In this tutorial, we are going to show you multiple ways in which you can convert any image into Grayscale in Python by using different libraries like Skimage, Pillow, OpenCV, Numpy, Matplotlib, and ImageIO. Each of the ways will be shown with examples for easy understanding.
Different Ways to Convert Image to Grayscale in Python
Input Image
For all the examples, the below dog image is going to be used as input.
1. Convert Image to Grayscale with Python Skimage (Scikit Image) – color.rgb2gray()
Scikit Image or Skimage is a Python based open-source package for various image processing algorithms. Any color image can be converted to grayscale with the help of color.rgb2gray() function of Skimage.
In the below example, the image is read using io.imread() and then it is converted to grayscale with color.rgb2gray() and finally it is displayed with io.imshow()
In [0]:
from skimage import color from skimage import io img = io.imread('dog.jpg') imgGray = color.rgb2gray(img) io.imshow(imgGray)
Out[0]:
<matplotlib.image.AxesImage at 0x7f4161961c90>
2. Convert Image to Grayscale with Python Skimage (Scikit Image) – io.imread()
There is another way to convert image to grayscale with Python Skimage, by using io.imread as shown below. While reading the image with io.imread we pas the parameter as_gray=True which converts the image to grayscale.
In [1]:
from skimage import io #convert image to grayscale img_gray = io.imread('dog.jpg', as_gray=True) io.imshow(img_gray)
Out[1]:
3. Image Grayscale Conversion with Pillow (PIL) – convert()
Pillow is another image processing library of Python that can be used to convert image to grayscale with its img.convert() function.
In this example, the image is read with Image.open() and then it is transformed with convert() by passing ‘L’ as the parameter. The ‘L’ parameter is used to convert the image to grayscale.
In [2]:
from PIL import Image img = Image.open('dog.jpg') imgGray = img.convert('L') imgGray.show()
Out[2]:
4. Image Grayscale Conversion with OpenCV – cv2.imread()
OpenCV is the most popular image processing package out there and there are a couple of ways to transform the image to grayscale. In this first approach, the image can be changed to grayscale while reading the image using cv2.imread() by passing the flag value as 0 along with the image file name.
In[3]:
import cv2 # Convert to Grayscale img_gray=cv2.imread("dog.jpg",0) # To display Image window_name='Grayscale Conversion OpenCV' cv2.namedWindow(window_name, cv2.WINDOW_NORMAL) cv2.imshow(window_name,img_gray) cv2.waitKey(0) cv2.destroyAllWindows()
Out[3]:
5. Image Grayscale Conversion with OpenCV – cv2.cvtColor()
In the next technique, the image can be changed to grayscale with the help of cv2.cvtColor() of the OpenCV module which is used for colorspace change.
The parameter to be used with this function for the grayscale is COLOR_BGR2GRAY as we can see in the below example.
In [4]:
import cv2 # Convert to Grayscale img_gray = cv2.cvtColor(img,cv2.COLOR_BGR2GRAY) # To display Image window_name='Grayscale Conversion OpenCV' cv2.namedWindow(window_name, cv2.WINDOW_NORMAL) cv2.imshow(window_name,img_gray) cv2.waitKey(0) cv2.destroyAllWindows()
Out[4]:
6. Convert Image to Grayscale with Python Numpy – 1st Approach
In this example, we will show how we can convert image to grayscale with Python Numpy library. At first, we read the image as a Numpy array with matplotlib plt.imread function. We can extract the red, green, and blue channels from the image. Finally, we multiply these individual channels with weights of ITU-R 601-2 luma transform and sum them up.
This produces the image in grayscale, as shown in the output.
In [5]:
import matplotlib
import matplotlib.pyplot as plt
import numpy as np
img = plt.imread('dog.jpg')
red = img[:, :, 0]
green = img[:, :, 1]
blue = img[:, :, 2]
# convert image to grayscale
gray_img = (0.299 * red + 0.587 * green + 0.114 * blue)
plt.imshow(gray_img, cmap=plt.get_cmap("gray"))
plt.axis('off')
plt.show()
Out[5]:
7. Convert Image to Grayscale with Python Numpy – 2nd Approach
In this example, we use another set of weights to convert image to grayscale with the Numpy operation as shown below.
In [6]:
import matplotlib
import matplotlib.pyplot as plt
import numpy as np
img = plt.imread('dog.jpg')
red = img[:, :, 0]
green = img[:, :, 1]
blue = img[:, :, 2]
# convert image to grayscale
gray_img = (0.2126 * red + 0.7152 * green + 0.0722 * blue)
plt.imshow(gray_img, cmap=plt.get_cmap("gray"))
plt.axis('off')
plt.show()
Out[6]:
8. Grayscale Conversion with ImageIO Library
ImageIo is a Python library that gives you an easy interface to read and write different types of image data. It can also be used for the grayscale conversion of Images as shown in the below example.
In [7]:
import imageio import matplotlib.pyplot as plt # Convert to grayscale using 'F' for floating-point or 'L' for integer-valued grayscale img_gray = imageio.imread('dog.jpg', pilmode='L') # Save the grayscale image imageio.imsave('dog_gray_imageio.jpg', img_gray)
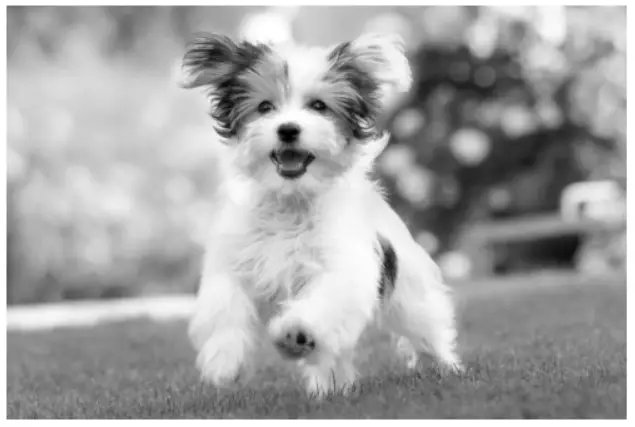