Introduction
OpenCV provides a method named cv2.cvtColor() which is used to convert an image from one color space to another. There are more than 150 color spaces are available in OpenCV. We will discuss the important ones in this article. Following Color spaces we are going to cover in this tutorials –
- Grayscale
- HSV Color Space
- RGB Color Space
- LAB Color Space
import cv2
Color space Conversion: cv2.cvtColor()
cvtColor() function is used to convert colored images to grayscale.
Syntax
cv2.cvtColor(src, code, dst, dstCn)
Parameters:
src: It is the image whose color space is to be changed.
code: It is the color space conversion code. It is basically an integer code representing the type of the conversion, for example, RGB to Grayscale.
Some codes are
cv2.COLOR_BGR2GRAY: This code is used to convert BGR colored image to grayscale
cv2.COLOR_BGR2HSV : This code is used to change the BGR color space to HSV color space.
cv2.COLOR_BGR2RGB : This code is used to change the BGR color space to RGB color space.
cv2. cv2.COLOR_BGR2LAB: This code is used to change the BGR color space to LAB color space.
dst: It is the output image of the same size and depth as src image. It is an optional parameter.
dstCn: It is the number of channels in the destination image. If the parameter is 0 then the number of the channels is derived automatically from src and code. It is an optional parameter.
Reading the Sample Image
# Reading the sample image
img=cv2.imread("image.jpg")
#Displaying the sample image
window_name='image'
cv2.namedWindow(window_name, cv2.WINDOW_NORMAL)
cv2.imshow(window_name,img)
cv2.waitKey(0)
cv2.destroyAllWindows()
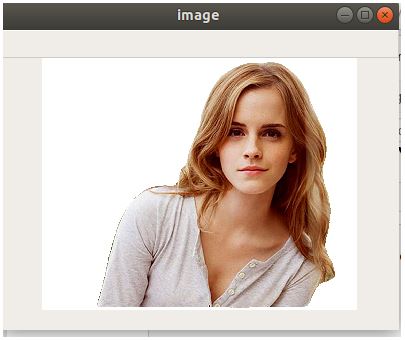
Conversion of BGR to Gray space using cv2.cvtColor() with code cv2.COLOR_BGR2GRAY
#Converting the image into grayscale
image = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY )
#Displaying the grayscale image
window_name='image_Gray'
cv2.namedWindow(window_name, cv2.WINDOW_NORMAL)
cv2.imshow(window_name,image)
cv2.waitKey(0)
cv2.destroyAllWindows()
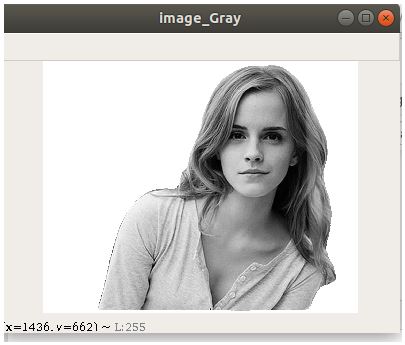
Conversion of BGR to HSV space using cv2.cvtColor() with code cv2.COLOR_BGR2HSV
The HSV color space has the following three components.
H – Hue ( Dominant Wavelength ).
S – Saturation ( shades of the color ).
V – Value ( Intensity ).
The best thing is that it uses only one channel to describe color (H), making it very intuitive to specify color.
#Converting image to HSV Space
image_HSV = cv2.cvtColor(img, cv2.COLOR_BGR2HSV )
#Displaying the HSV image
window_name='image_HSV'
cv2.namedWindow(window_name, cv2.WINDOW_NORMAL)
cv2.imshow(window_name,image_HSV)
cv2.waitKey(0)
cv2.destroyAllWindows()
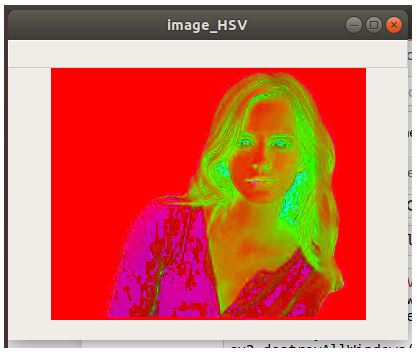
[adrotate banner=”3″]
Conversion of BGR to RGB space using cv2.cvtColor() with code cv2.COLOR_BGR2RGB
The RGB colorspace has the following properties –
- It is an additive colorspace where colors are obtained by a linear combination of Red, Green, and Blue values.
- The three channels are correlated by the amount of light hitting the surface.
#Converting image to RGB Space
image_RGB = cv2.cvtColor(img, cv2.COLOR_BGR2RGB )
#Displaying the RGB Image
window_name='image_RGB'
cv2.namedWindow(window_name, cv2.WINDOW_NORMAL)
cv2.imshow(window_name,image_RGB)
cv2.waitKey(0)
cv2.destroyAllWindows()
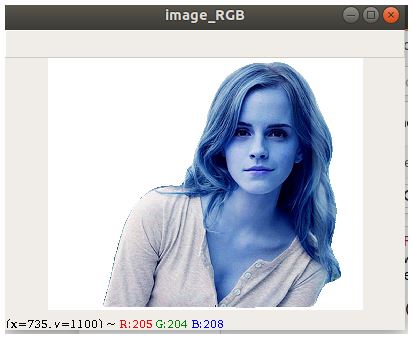
Conversion of BGR to LAB space using cv2.cvtColor() with code cv2.COLOR_BGR2LAB
The Lab color space has three components.
- L – Lightness ( Intensity ).
- a – color component ranging from Green to Magenta.
- b – color component ranging from Blue to Yellow.
The Lab color space is quite different from the RGB color space.
In RGB color space the color information is separated into three channels but the same three channels also encode brightness information.
On the other hand, in Lab color space, the L channel is independent of color information and encodes brightness only. The other two channels encode color.
#Converting image to LAB Space
image_LAB = cv2.cvtColor(img, cv2.COLOR_BGR2LAB )
#Displaying the LAB image
window_name='image_LAB'
cv2.namedWindow(window_name, cv2.WINDOW_NORMAL)
cv2.imshow(window_name,image_LAB)
cv2.waitKey(0)
cv2.destroyAllWindows()
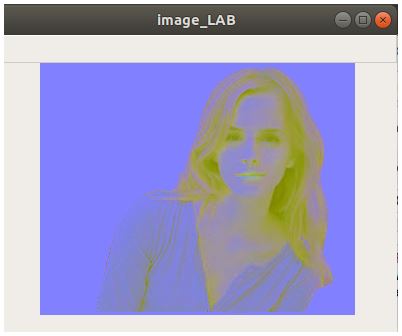
Conclusion
So in this article, we saw how we can change the color of an image to various color spaces using cvtcolor() function of OpenCV. We converted a sample image into grayscale, HSV, RGB and LAB color space.
Reference – https://docs.opencv.org/master/d6/d00/tutorial_py_root.html
-
My name is Sachin Mohan, an undergraduate student of Computer Science and Engineering. My area of interest is ‘Artificial intelligence’ specifically Deep learning and Machine learning. I have attended various online and offline courses on Machine learning and Deep Learning from different national and international institutes My interest toward Machine Learning and deep Learning made me intern at ISRO and also I become the 1st Runner up in TCS EngiNX 2019 contest. I always love to share my knowledge and experience and my philosophy toward learning is "Learning by doing". So thats why I believe in education which have include both theoretical as well as practical knowledge.
View all posts