Introduction
Images may contain various types of noises that reduce the quality of the image. Blurring or smoothing is the technique for reducing the image noises and improve its quality. Usually, it is achieved by convolving an image with a low pass filter that removes high-frequency content like edges from the image. In this tutorial, we will see methods of Averaging, Gaussian Blur, and Median Filter used for image smoothing and how to implement them using python OpenCV, built-in functions of cv2.blur(), cv2.GaussianBlur(), cv2.medianBlur().
Importing OpenCV Library
import cv2
Image used for this Tutorial
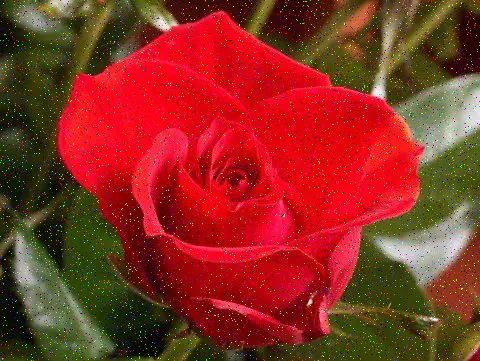
Averaging: cv2.blur()
Note: The smoothing of an image depends upon the kernel size. If Kernel size is large then it removes the small feature of the image. But if the kernel size is too small then it is not able to remove the noise.
Syntax
cv2.blur(src, ksize, dst, anchor, borderType)
src: It is the image whose is to be blurred.
ksize: A tuple representing the blurring kernel size.
dst: It is the output image of the same size and type as src.
anchor: It is a variable of type integer representing anchor point and it’s default value Point is (-1, -1) which means that the anchor is at the kernel center.
borderType: It depicts what kind of border to be added. It is defined by flags like cv2.BORDER_CONSTANT, cv2.BORDER_REFLECT, etc
Return Value: It returns an image.
Example of Smoothing Image using cv2.blur()
#reading an image
img_first=cv2.imread("noiseimage.jpg")
#We are taking Kernel Size as 5x5
img_blur = cv2.blur(img_first,(5,5))
#Display blur image
window_name='image_blur'
cv2.namedWindow(window_name, cv2.WINDOW_NORMAL)
cv2.imshow(window_name,img_blur)
cv2.waitKey(0)
cv2.destroyAllWindows()
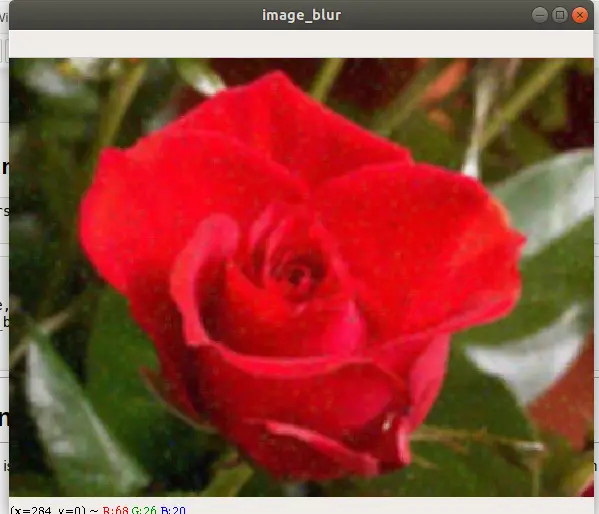
[adrotate banner=”3″]
Gaussian Blur: cv2.GaussianBlur()
Syntax
cv2.GaussianBlur( src, dst, size, sigmaX, sigmaY = 0, borderType =BORDER_DEFAULT)
src It is the image whose is to be blurred.
dst output image of the same size and type as src.
ksize Gaussian kernel size. ksize.width and ksize.height can differ but they both must be positive and odd.
sigmaX Gaussian kernel standard deviation in X direction.
sigmaY Gaussian kernel standard deviation in Y direction; if sigmaY is zero, it is set to be equal to
sigmaX, if both sigmas are zeros, they are computed from ksize.width and ksize.height, respectively
borderType: Specifies image boundaries while kernel is applied on image borders.
Possible values are: cv2.BORDER_CONSTANT cv2.BORDER_REPLICATE cv2.BORDER_REFLECT cv2.BORDER_WRAP cv2.BORDER_REFLECT_101 cv2.BORDER_TRANSPARENT cv2.BORDER_REFLECT101 cv2.BORDER_DEFAULT cv2.BORDER_ISOLATED
Example of Smoothing Image using cv2.GaussianBlur()
#Read Image
img_second=cv2.imread("noiseimage.jpg")
In [6]:
#We are taking Kernel size as 5x5
gaussian_blur = cv2.GaussianBlur(img_second,(5,5),sigmaX=0)
#Display gaussian blur image
window_name='imagesecond_blur'
cv2.namedWindow(window_name, cv2.WINDOW_NORMAL)
cv2.imshow(window_name,gaussian_blur)
cv2.waitKey(0)
cv2.destroyAllWindows()
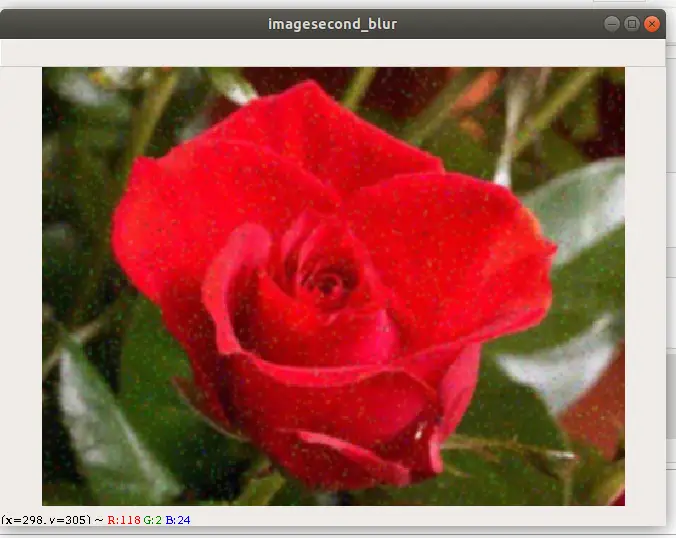
Median Filter: cv2.medianBlur()
Note: This is highly effective in removing salt-and-pepper noise.
Syntax
cv2.medianBlur( src,dst,ksize )
src : It is the image that is to be blurred.
dst : destination array of the same size and type as src.
ksize : aperture linear size; it must be odd and greater than 1, for example 3, 5, 7 …
Example of Smoothing Image using cv2.medianBlur()
img_third=cv2.imread("noiseimage.jpg")
image_median = cv2.medianBlur(img_third,5)
Median Blurring always reduces the noise effectively because in this filtering technique the central element is always replaced by some pixel value in the image. But in the above filters, the central element is a newly calculated value which may be a pixel value in the image or a new value.
#Display MedianBlurred image
window_name='MedianBlurrred'
cv2.namedWindow(window_name, cv2.WINDOW_NORMAL)
cv2.imshow(window_name,image_median)
cv2.waitKey(0)
cv2.destroyAllWindows()
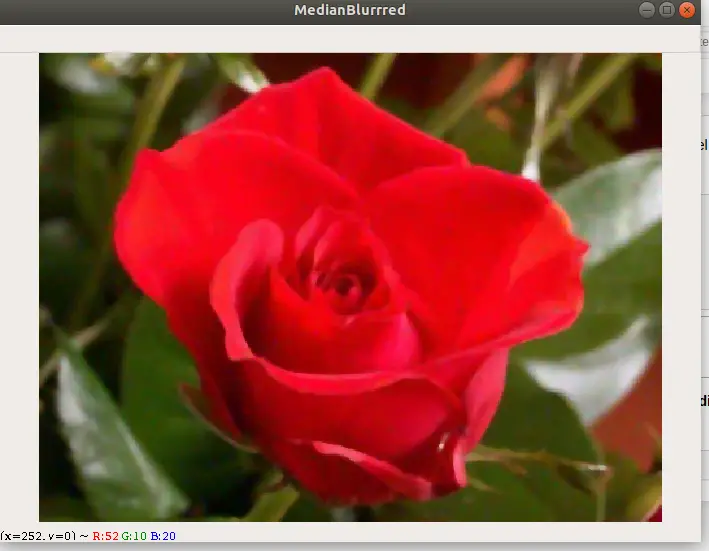
As you can see here the salt pepper noise gets drastically reduced using cv2.medianBlur() OpenCV function
Conclusion
Reaching the end of this tutorial, we learned image smoothing techniques of Averaging, Gaussian Blur, and Median Filter and their python OpenCV implementation using cv2.blur() , cv2.GaussianBlur() and cv2.medianBlur().
Reference – https://docs.opencv.org/master/d6/d00/tutorial_py_root.html
-
My name is Sachin Mohan, an undergraduate student of Computer Science and Engineering. My area of interest is ‘Artificial intelligence’ specifically Deep learning and Machine learning. I have attended various online and offline courses on Machine learning and Deep Learning from different national and international institutes My interest toward Machine Learning and deep Learning made me intern at ISRO and also I become the 1st Runner up in TCS EngiNX 2019 contest. I always love to share my knowledge and experience and my philosophy toward learning is "Learning by doing". So thats why I believe in education which have include both theoretical as well as practical knowledge.
View all posts