Introduction
In this tutorial, we will learn how to create zero tensor in PyTorch by using torch.zeros() and torch.zeros_like() functions. Zero tensors are tensors whose all values are zero as shown in the below illustration.
This example shows 2 dimensional zero tensors of size 2×2, 3×3, and 2×3.
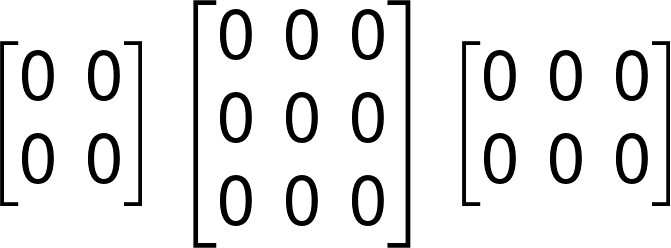
PyTorch Zeros Tensors with torch.zeros()
You can easily create Tensors with all zeros in PyTorch by using torch.zeros function. Let us understand this function with the help of a few examples. But before that, we have to import the PyTorch library as shown below –
In [0]:
import torch;
Example – 1 : Creating 2 Dimensional Zero Tensor with torch.zeros()
In the first example, we are creating a zero tensor of size 3×5. For this we pass this size as a list in torch.zeros function as shown below.
In [1]:
zero_tensor = torch.zeros(size = [3,5]) zero_tensor
Out[1]:
tensor([[0., 0., 0., 0., 0.], [0., 0., 0., 0., 0.], [0., 0., 0., 0., 0.]])
zero_tensor = torch.zeros(size = (3,5)) zero_tensor
Out[2]:
tensor([[0., 0., 0., 0., 0.], [0., 0., 0., 0., 0.], [0., 0., 0., 0., 0.]])
Example – 2 : Creating Zero Tensor with Specific Data Type
By default, the torch zeros function creates the tensor with data type float. This is why the zero tensors in the above examples had zeros with decimals. We can specify the data type explicitly by using dtype parameter.
In the below example, we are passing dtype as int and in output, we can see all zeros are of type int without any decimal points.
zero_tensor = torch.zeros(size = (3,5), dtype =int) zero_tensor
tensor([[0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0]])
Example – 3 : Creating 3 Dimensional Zero Tensor with torch.zeros()
In this example, we are creating 3-dimensional zeros tensor in PyTorch as shown below.
In [4]:
zero_tensor = torch.zeros(size = (2,3,5), dtype =int) zero_tensor
Out[4]:
tensor([[[0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0]], [[0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0]]])
PyTorch Zeros Tensors with torch.zeros_like()
Example – 1 : Creating 2 Dimensional Zero Tensor with torch.zeros_like()
Let us first create a tensor with random values whose size will be used for creating the zeros tensor. We pass the name of this tensor to torch.zeros_like function
In [5]:
random_tensor = torch.rand(size=(4,5)) random_tensor
Out[5]:
tensor([[0.0974, 0.5907, 0.5231, 0.1925, 0.2946], [0.7735, 0.1797, 0.0895, 0.4779, 0.9663], [0.0556, 0.1394, 0.0433, 0.9831, 0.3034], [0.8552, 0.9015, 0.6390, 0.6234, 0.9691]])
zero_like_tensor = torch.zeros_like(random_tensor,dtype =int) zero_like_tensor
Out[6]:
tensor([[0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0]])
Example – 2 : Creating 3 Dimensional Zero Tensor with torch.zeros_like()
Again, let us create a 3-D random valued tensor of size 2x4x5 and then use it in torch.zeros_like function.
In [7]:
random_tensor = torch.rand(size=(2,4,5)) random_tensor
Out[7]:
tensor([[[0.0702, 0.4700, 0.8626, 0.5981, 0.2569], [0.5060, 0.4742, 0.7492, 0.3597, 0.8754], [0.7581, 0.8954, 0.3205, 0.7038, 0.3167], [0.1799, 0.9890, 0.9624, 0.2595, 0.6935]], [[0.0338, 0.9996, 0.2049, 0.0127, 0.1300], [0.6330, 0.4086, 0.6630, 0.0960, 0.5759], [0.1510, 0.3219, 0.5052, 0.5823, 0.8093], [0.1546, 0.9815, 0.6459, 0.1247, 0.1438]]])
zero_like_tensor = torch.zeros_like(random_tensor,dtype =int) zero_like_tensor
tensor([[[0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0]], [[0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0]]])